DisposableSignature provider
Bulksign supports a generic "disposable/one shot" signature that allows signing integration with a remote Certification Authority. The following integration scenarios are possible :
-
the remote Certification Authority emits a new short/medium lived certificate which can be used for the current signing session.
-
sign with an existing long term individual certificate kept on the CA HSM.
Signing Flow
Here is a short high level overview of the signing process from the signer perspective :
-
after initiating the signing process, the signer is presented with the CertificationAuthority certificate issuing agreement.
-
signer accepts the agreement
-
an OTP is sent to the user (usually by SMS) by the CertificationAuthority to identify the user
-
user enters the OTP for validation. If the OTP is correct ,the signing process proceeds.
Provider Overview
The provider definition from Bulksign Extensibility looks like this :
public interface IDisposableSignProvider : ISignProvider { OperationResult VerifySigner(SignerDetails signerDetails,Dictionary<string,string> options); DisposableOtpResult SendOtp(DisposableSendOtp otp, SignerDetails signerDetails,Dictionary<string,string> options); OperationResult ValidateOtp(DisposableValidateOtp otp,SignerDetails signerDetails,Dictionary<string,string> options); SignedHashResult SignHash(byte[] hash,SignerDetails signerDetails,Dictionary<string,string> options); IssuanceAgreementResult GetIssuanceAgreement(SignerDetails signerDetails,Dictionary<string,string> options); DisposableSignatureResult GetSignatureImage(SignerDetails signerDetails,int signatureHeight, int signatureWidth, Dictionary<string,string> options); SignerField[] Fields { get; set; } bool ProvidesSignatureImage { get; } bool RequiresIssuerAgreementAcceptance { get; } bool IsPhoneNumberRequiredForSigner { get; } int OtpValiditySeconds { get; } string OtpLocalizationKey { get; set; } string PublicKeyBase64 { get; } }
Here is the meaning of each property :
-
Fields : set the provider's required fields, which can be edited by the envelope sender from the UI.
-
RequiresIssuerAgreementAcceptance : flag which determines if the signer is required to accept the certificate issuance agreement before signing.
-
IsPhoneNumberRequiredForSigner : flag which determines if the signer needs to have the phone number assigned. If the integration requires the OTP to be sent by SMS, please set this to true.
-
ProvidesSignatureImage (optional) : set this to true if the provider needs to provide the image of the signature.
-
OtpValiditySeconds : the vality of the OTP in seconds.
-
OtpLocalizationKey (optional) : set the name of the localization key which contains the SMS text for sending the OTP. If this key is set, the value will be passed on method SendOtp.
-
SignatureName : the name of the signature provider which will be shown in the UI.
-
PublicKeyBase64 : the public key of the signing certificate in base64 format. For disposable certificate, just use the public key of the root certificate.
-
SignatureIdentifier : the internal signature identifier. If multiple remote signature providers are implemented just assign each one a different number starting from 100.
Methods order flow
The order in which the methods are invoked is :
-
VerifySigner : this is invoked when the envelope is sent and it allows you to validate the signer information. If the information is invalid/wrong please return an error message that indicates what field(s) are invalid.
-
GetIssuanceAgreement (optional) : is invoked when the issuer agreement is required to be shown to the signer.
-
SendOtp : is invoked after the signer accepts the issuer agreement.
-
ValidateOtp : is invoked after the user enters the OTP.
-
GetSignatureImage (optional) : if the provider is configured to return the signature image, is invoked after the OTP is successfully validated.
-
SignHash : is invoked last when all preconditions are met and oes the actual signing.
Notes
-
the signer certficate issuer agreement is only shown once per signing session.
-
the signer cannot request a new OTP (which is configured with OtpValiditySeconds) to be sent until the current OTP request expires.
FAQ
How do I use this provider from Bulksign UI ?
If the provider is registered, the new signature type will appear in Bulksign UI along with the rest of the signature types.
How can the envelope sender edit the required provider fields ?
Each recipient has an option for editing the fields for each registered provider. Here is how it looks for the demo sample provider :
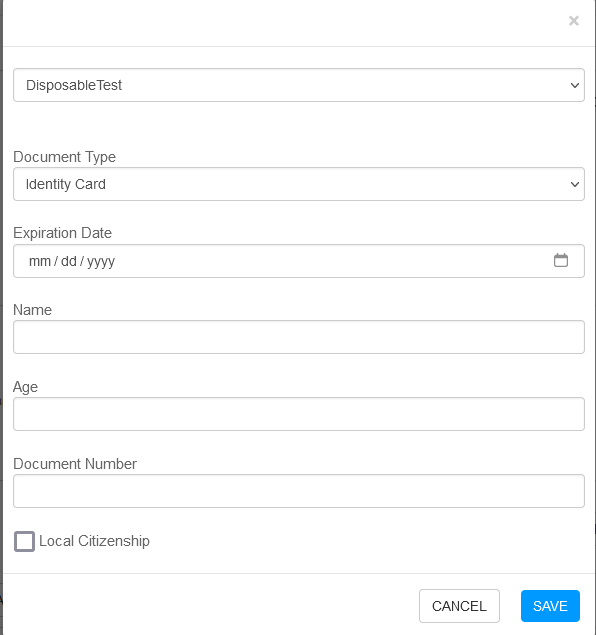
Is there a sample which demonstrates implementing a disposable signing provider ?
Please see this sample code on Github