How do I ?
Work with temporary files ?
Bulksign API allows you to upload 1 or more PDF files once and "reference" them when calling SendEnvelope multiple times.
This is done by calling the "StoreTemporaryFile" API method. The method returns a file identifier which can be used when calling SendEnvelope :
ApiResult<string> resultApiModel = api.StoreTemporaryFile(....); EnvelopeApiModel newEnvelope = new EnvelopeApiModel(); newEnvelope.Documents = new[] { new DocumentApiModel() { FileIdentifier = new FileIdentifier() { Identifier = resultApiModel.Response }, FileName = "contract.pdf" } };
In the SAAS version of Bulksign, the files can be referenced for up to 30 minutes after upload. For on premise version, this is configurable, the setting is called
TimeToKeepTemporaryFilesInMinutes
How can I find the metadata for my PDF files (form field names) to use for field mapping ?
This can be done dynamically with the "AnalyzeFile" API method. That will return a FormFieldResultApiModel[] which contain the following info :
Id - the form field identifier
PageNumber - the page number where the form field is located
FieldType - the type of the form field
How do I send PDF files ?
We support multiple ways to pass PDF files. Each method is designed for different integration scenarios :
- pass the file content directly in the API request.
This is designed for the scenario where each request to SendEnvelope contains unique PDF files. The input supports passing the file's content as both byte array and base64 encoded strings.
Example as byte[]:
DocumentApiModel doc = new DocumentApiModel(); doc.FileContentByteArray = new FileContentByteArray() { ContentBytes = File.ReadAllBytes(@"c:\contract.pdf") };
Example as bas64 string :
DocumentApiModel doc = new DocumentApiModel(); doc.FileContentBase64String = new FileContentBase64String() { FileContent = Convert.ToBase64String(File.ReadAllBytes(@"c:\contract.pdf")) };
- pass a file reference
This is designed for the scenario where same file needs to be signed and can be "reused" between multiple SendEnvelope requests. See also working with temporary files
Example :
ApiResult<string> resultApiModel = app.StoreTemporaryFile(....); EnvelopeApiModel newEnvelope = new EnvelopeApiModel(); newEnvelope.Documents = new[] { new DocumentApiModel() { FileIdentifier = new FileIdentifier() { Identifier = resultApiModel.Response }, FileName = "contract.pdf" } };
- pass the path to the file (OnPremise version only)
This is designed for the scenario where you integrate Bulksign in a a heterogenous on-premise environment and you can pass directly the path (either local or network share) to the PDF file generated by another application.
Example :
newEnvelope.Documents = new [] { new DocumentApiModel() { FileNetworkShare = new FileNetworkShare() { Path = "\\docshare\contract.pdf" } } };
Sample code is available here
How automatic field assignment works when creating drafts/templates/envelopes ?
Automatic field assignment works depending on the number of recipients per envelope:
-
for 1 recipient, unless FieldAssignments is specified, all existing form fields will be, by default, assigned to that recipient.
-
for bulk envelopes , unless FieldAssignments is specified, all existing fields will be assigned, by default, to all bulk recipients.
How do I assign fields already existing in the document to different recipients :
This is done with the FieldAssignments array. For each recipient, add a new FieldAssignment with following info :
AssignedToRecipientEmail : this is the recipient mapping identifier and can have one of these 3 values : - the '*' character to assign that particular field to all recipients (usable for bulk envelopes). - the email address of the recipient to whom the field will be assigned. - the name of the recipient (this should be used only in the scenario of disabling email notifications for multiple recipients)
Fields or Signatures array must be populated with the form fields/ signature fields you want to assign.
Form field have these properties :
FieldId : the id of the form field which will be assigned
IsRequired : required flag (if the form field type supports it)
Signature fields have the following properties :
FieldId : id of the signature field
SignatureType : the type of the signature field
Sample code on Github here
How can I add a new signature field from the API ?
There are two options to add new signature fields to documents :
-
add a special text in the document (called tag) which will be converted to a signature field. Documentation is here
-
specify the position of the signature in the page directly from the API :
You can accomplish this by populating the "NewSignatures" array. The values for "NewSignatureApiModel" :
Width : the width of the signature field (in pixels).
Height : the height of the signature field (in pixels).
Top : the position (in pixels) from the top side of the page.
Left : the position (in pixels) from the left side of the page.
PageIndex : the index of page to which this new signature will be added.
AssignedToRecipientEmail : use the recipient email address to assign this to a specific recipient or character '*' to assign field to all recipients (in bulk scenarios). In case the email address is noreply@bulksign.com, use the recipient's name.
Sample code on Github here
How can I found out if a envelope has rejected signing steps ?
This can be done by checking the "Status" of each of the signer recipients. Additionally, if the status is "Rejected" (3) , "RejectionMessage" will contain the message from the signer.
How can I know if signing has been delegated to a certain recipient ?
The flag is called "HasBeenDelegated", if is set to true it means the current recipient has been delegated to sign.
With a concurrent envelope, how do i find out which recipient locked access to signing the envelope ?
Call GetEnvelopeDetails and iterate through all recipients. The recipient which has a non null value in "LockedDate" means is the recipient which locked signing access.
How can I add text annotations to documents ?
Please see the full sample code on Github Here's some sample code for this :
BulksignNewAnnotation annCustom = new BulksignNewAnnotation { Height = 300, PageIndex = 1, Left = 10, Top = 650, FontSize = 28, Type = BulksignAnnotationType.Custom, CustomText = "Annotation with custom text spaning multiple lines of text because the text is too long" }; BulksignNewAnnotation annSenderName = new BulksignNewAnnotation { Height = 100, PageIndex = 1, Left = 10, Top = 900, FontSize = 28, Type = BulksignAnnotationType.SenderName }; BulksignNewAnnotation annOrganization = new BulksignNewAnnotation { Height = 100, PageIndex = 1, Left = 10, Top = 940, FontSize = 28, Type = BulksignAnnotationType.OrganizationName };
There are 4 types of annotations supported :
- Custom : allows you to enter a custom text
- OrganizationName : will output the name of the Bulksign organization from which the envelope is sent
- SenderName : will output the name of the sender
- SenderEmail : will output the email of the sender
The position of the text in the page is done with Top/Left properties, while "Height" sets the vertical size of the text. For long text that you expect to wrap on multiple lines, please set a high height value (like shown in the sample text above).
Here is how the text annotations look like when applied to the pdf :
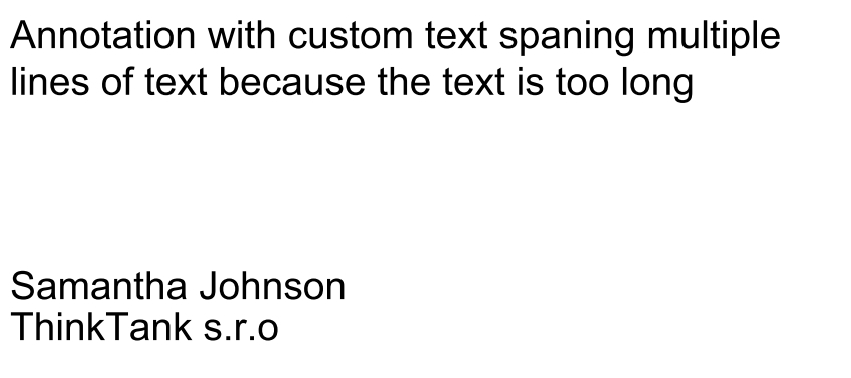
Is there a easy way to determine the position (top and left) of the new form fields on the page ?
Yes, take a screenshot of the pdf document page (or obtained from a Bulksign draft) and open it in a graphics editor application (something like Paint.net for example) . Make sure the ruler feature is enabled , and the mouse cursor position should be shown in the status bar. Just move the mouse cursor where you would like to position the form field and save the x,y values.
What APIs can be used with global authentication key ?
SendEnvelopeFromDraft
SendEnvelopeFromTemplate
GetEnvelopeStatus
GetEnvelopeDetails
DownloadEnvelopeCompletedDocuments
DownloadEnvelopeIncompleteDocuments
RestartEnvelope
CancelEnvelope
SendReminder
DeleteEnvelopeRecipient
ReplaceEnvelopeRecipient
UpdateDraftSettings
AddDocumentsRecipientsToDraft
DeleteDraft
GetTemplateDetails
UpdateTemplateSettings
AddDocumentsRecipientsToTemplate
DeleteTemplate
GetCompletedFormFields
DeleteEnvelope
UnlockConcurrentRecipient
Which fields SearchEnvelopes/Drafts/Templates is searching ?
The Search* methods (for own and team members documents) will search the following fields :
- name
- email subject
- recipient emails
- recipient name
How can I find out which PDF attachments were attached by which signer?
Call GetEnvelopeDetails and iterate through all recipients. Each recipient has a "Attachments" property which returns the attachment identifier and the PDF filename to which the attachment was added. Sample code which demonstrates how to add attachment requests to an envelope is available on GitHub